7 Developer Reference
None of these APIs should be considered stable enough for use in projects other than Frosthaven Manager. They should be considered stable enough for use in Frosthaven Manager. Changes to an internally-used API should be made with care and compelling reason.
7.1 aoe
This module implements the Area-of-Effect (AoE) language. See Programming a Scenario and frosthaven-manager/aoe for more information.
7.2 aoe-images
(require frosthaven-manager/aoe-images) | |
package: frosthaven-manager |
This module provides procedures for constructing area-of-effect diagrams.
parameter
(hex-size size) → void? size : natural-number/c
= 30
procedure
(border-size max-row max-col) → (and/c positive? number?)
max-row : natural-number/c max-col : natural-number/c
value
spec-sym? : flat-contract? = (or/c 's 'x 'o 'm 'g)
value
=
(listof (list/c exact-positive-integer? boolean? (listof (list/c spec-sym? natural-number/c))))
procedure
(spec->shape s) → pict?
s : spec?
The symbols represent the corresponding shapes, with 'g a ghost hex.
value
procedure
(syntaxes->spec stxs) → spec?
stxs : (and/c (listof syntax?) syntaxes-can-be-spec?)
procedure
(string->spec s) → spec?
s : string?
7.3 Constant Formatting and Parsing
(require frosthaven-manager/constants) | |
package: frosthaven-manager |
This module provides forms for binding formatters and parsers where the mapping from constant to string and vice-versa is static.
syntax
(define-constant-format/parse formatter-id parser-id ([constant-id string] ...))
syntax
(define-constant-format formatter-id ([constant-id string] ...))
syntax
(define-constant-parse parser-id ([constant-id string] ...))
7.4 contracts
(require frosthaven-manager/contracts) | |
package: frosthaven-manager |
procedure
(unique-with/c key c) → contract?
key : (-> any/c any/c) c : flat-contract?
7.5 curlique
(require frosthaven-manager/curlique) | |
package: frosthaven-manager |
This module provides a shorthand notation for Qi flows. It overrides Racket’s #%app: forms written in curly braces like {(all positive?)} are implictly wrapped in flow from Qi.
In addition, it provides the following overrides:
; A very small identity > (map {} (range 10)) '(0 1 2 3 4 5 6 7 8 9)
> (define all-good? {(all positive?)}) > (all-good? 1 2 3 4) #t
> (all-good? 1 -2 3 4) #f
> (~> (1 2 3) (-< + count) /) 2
> (define average {~> (-< + count) /}) > (average 1 2 3) 2
7.6 defns
(require frosthaven-manager/defns) | |
package: frosthaven-manager |
This module reprovides everything from frosthaven-manager/defns/level, frosthaven-manager/defns/loot, frosthaven-manager/defns/monsters, frosthaven-manager/defns/players, and frosthaven-manager/defns/scenario.
7.6.1 Level Info
(require frosthaven-manager/defns/level) | |
package: frosthaven-manager |
struct
(struct level-info ( monster-level gold trap-damage hazardous-terrain exp) #:transparent) monster-level : natural-number/c gold : natural-number/c trap-damage : natural-number/c hazardous-terrain : natural-number/c exp : natural-number/c
value
value
value
value
procedure
(get-level-info level) → level-info?
level : level/c
procedure
(inspiration-reward num-players) → natural-number/c
num-players : num-players/c
7.6.2 Loot Deck
(require frosthaven-manager/defns/loot) | |
package: frosthaven-manager |
value
value
value
value
value
Serializable.
value
value
value
value
value
value
value
value
Serializable.
value
value
Serializable.
struct
(struct money (amount) #:transparent) amount : natural-number/c
Serializable.
struct
(struct material (name amount) #:transparent) name : material-kind? amount : (apply list/c (make-list (sub1 max-players) natural-number/c))
Serializable.
procedure
(material-amount* m n) → natural-number/c
m : material? n : num-players/c
struct
(struct herb (name amount) #:transparent) name : herb-kind? amount : natural-number/c
Serializable.
struct
(struct special-loot (name) #:transparent) name : string?
Serializable.
value
= (or/c money? material? herb? random-item? special-loot?)
value
= (or/c 'money material-kind? herb-kind? 'random-item 'special)
procedure
(card->type c) → loot-type/c
c : loot-card?
procedure
((format-loot-card n) card) → string?
n : num-players/c card : loot-card?
value
value
value
value
value
money-deck : (apply list/c (make-list max-money-cards money?))
value
: (hash/c material-kind? (apply list/c (make-list max-material-cards material?)))
value
: (hash/c herb-kind? (apply list/c (make-list max-herb-cards herb?)))
value
standard-loot-deck : (hash/c loot-type/c (listof loot-card?))
procedure
(apply-sticker card) → loot-card?
card :
(and/c loot-card? (not/c random-item?) (not/c special-loot?))
7.6.3 Monster Cards
(require frosthaven-manager/defns/monsters) | |
package: frosthaven-manager |
struct
(struct monster-stats ( max-hp move attack bonuses effects immunities) #:prefab) max-hp : (or/c positive-integer? string?) move : (or/c #f natural-number/c) attack : (or/c natural-number/c string?) bonuses : (listof string?) effects : (listof string?) immunities : (listof string?)
struct
(struct monster-info (set-name name normal-stats elite-stats) #:prefab) set-name : string? name : string? normal-stats : (apply list/c (make-list number-of-levels monster-stats?)) elite-stats : (apply list/c (make-list number-of-levels monster-stats?))
struct
(struct monster-ability ( set-name name initiative abilities shuffle?) #:prefab) set-name : string? name : string? initiative : initiative? abilities : (listof (listof (or/c string? pict?))) shuffle? : boolean?
Note that pre-fab syntax does not permit path? or pict? objects.
The monster abilities are a list of sub-abilities, much like a character’s ability card. Each sub-ability is a list of parts, which may include pictures such as for embedded AoE diagrams.
value
struct
(struct monster (number elite? current-hp conditions) #:transparent) number : monster-number/c elite? : boolean? current-hp : natural-number/c conditions : (listof condition?)
Prefer the smart constructor make-monster.
Serializable.
struct
(struct monster-group ( set-name name level normal-stats elite-stats monsters) #:transparent) set-name : string? name : string? level : level/c normal-stats : monster-stats? elite-stats : monster-stats? monsters : (listof monster?)
Prefer the smart constructor make-monster-group and the update functions, which maintain an invariant of monsters sorted by eliteness and number.
Serializable.
procedure
(monster-stats-max-hp* stats env) → positive-integer?
stats : monster-stats? env : env/c
procedure
(monster-stats-attack* stats env) → positive-integer?
stats : monster-stats? env : env/c
procedure
m : monster-stats?
procedure
m : monster-stats?
procedure
m : monster-stats?
procedure
(monster-ability-name->text ability) → string?
ability : (or/c #f monster-ability?)
procedure
(monster-ability-initiative->text ability) → string?
ability : (or/c #f monster-ability?)
procedure
(monster-ability-ability->rich-text ability-text mg env) → (listof (or/c string? pict? pict/alt-text? newline?)) ability-text : (listof (or/c string? pict?)) mg : monster-group? env : env/c
procedure
(make-monster info level number elite? env) → monster?
info : monster-info? level : level/c number : monster-number/c elite? : boolean? env : env/c
procedure
(make-monster-group info level num+elite?s env) → monster-group? info : monster-info? level : level/c
num+elite?s :
(and/c (listof (cons/c monster-number/c boolean?)) (unique-with/c car any/c)) env : env/c
The num+elite?s parameter provides a mapping from (unique) monster numbers to their elite status. Only monster numbers in the mapping are added to the monster-group.
Formulas are calculated using env.
procedure
(get-monster-stats mg m) → monster-stats?
mg : monster-group? m : monster?
procedure
(monster-at-max-health? m s env) → boolean?
m : monster? s : monster-stats? env : env/c
procedure
(monster-dead? m) → boolean?
m : monster?
procedure
((monster-update-condition c on?) m) → monster?
c : condition? on? : boolean? m : monster?
procedure
m : monster?
procedure
((monster-group-update-num n f) mg) → monster-group?
n : monster-number/c f : (-> monster? monster?) mg : monster-group?
procedure
((monster-group-remove n) mg) → monster-group?
n : monster-number/c mg : monster-group?
procedure
((monster-group-add n elite? env) mg) → monster-group?
n : monster-number/c elite? : boolean? env : env/c mg : monster-group?
procedure
(monster-group-first-monster mg) → (or/c #f monster-number/c)
mg : monster-group?
procedure
(monster-group-update-level mg info new-level) → monster-group? mg : monster-group? info : monster-info? new-level : level/c
procedure
(monster->hp-text m ms env) → string?
m : monster? ms : monster-stats env : env/c
procedure
mg : monster-group?
procedure
(swap-monster-elite m) → monster?
m : monster?
procedure
(monster-group-change-max-HP mg f env) → monster-group?
mg : monster-group? f : (-> (or/c 'normal 'elite) natural-number/c number?) env : env/c
7.6.4 Players
(require frosthaven-manager/defns/players) | |
package: frosthaven-manager |
struct
(struct player ( name max-hp current-hp xp conditions initiative loot summons) #:transparent) name : string? max-hp : positive-integer? current-hp : natural-number/c xp : natural-number/c conditions : (listof condition?) initiative : initiative? loot : (listof loot-card?) summons : (listof summon?)
You will not usually need the player constructor: use the smart constructor make-player instead.
Serializable.
procedure
(make-player name max-hp) → player?
name : string? max-hp : positive-integer?
procedure
((player-update-name new-name) p) → player?
new-name : string? p : player?
procedure
((player-act-on-hp f) p) → player?
f : (-> natural-number/c number?) p : player?
procedure
((player-act-on-max-hp f) p) → player?
f : (-> natural-number/c number?) p : player?
procedure
((player-act-on-xp f) p) → player?
f : (-> natural-number/c number?) p : player?
procedure
((player-add-condition c) p) → player?
c : condition? p : player?
procedure
((player-remove-condition c) p) → player?
c : condition? p : player?
procedure
((player-condition-handler c?) p) → player?
c? : (list/c condition? boolean?) p : player?
procedure
((player-afflicted-by? c) p) → boolean?
c : condition? p : player?
procedure
p : player?
procedure
(player-dead? p) → boolean?
p : player?
In practice, player HP does not currently fall below 1. This may be a bug.
procedure
p : player?
procedure
(player-set-initiative p i) → player?
p : player? i : initiative?
procedure
p : player?
procedure
((player-add-loot card) p) → player?
card : loot-card? p : player?
procedure
(player->hp-text p) → string?
p : player?
procedure
(player-conditions* p) → (listof condition?)
p : player?
struct
(struct summon (name max-hp current-hp conditions) #:transparent) name : string? max-hp : positive-integer? current-hp : natural-number/c conditions : (listof condition?)
procedure
((summon-update-name new-name) s) → summon?
new-name : string? s : summon?
procedure
((summon-act-on-hp f) s) → summon?
f : (-> natural-number/c number?) s : summon?
procedure
((summon-act-on-max-hp f) s) → summon?
f : (-> natural-number/c number?) s : summon?
procedure
((summon-add-condition c) s) → summon?
c : condition? s : summon?
procedure
((summon-remove-condition c) s) → summon?
c : condition? s : summon?
procedure
((summon-condition-handler c?) s) → summon?
c? : (list/c condition? boolean?) s : summon?
procedure
((summon-afflicted-by? c) s) → boolean?
c : condition? s : summon?
procedure
(summon-dead? s) → boolean?
s : summon?
In practice, summon HP does not currently fall below 1. This may be a bug.
procedure
s : summon?
procedure
(summon->hp-text s) → string?
s : summon?
procedure
(summon-conditions* s) → (listof condition?)
s : summon?
procedure
(player-summon p name max-hp) → player?
p : player? name : string? max-hp : positive-integer?
procedure
((update-player-summon i f) p) → player?
i : natural-number/c f : (-> summon? summon?) p : player?
procedure
((player-kill-summon i) p) → player?
i : natural-number/c p : player?
7.6.5 Scenario
(require frosthaven-manager/defns/scenario) | |
package: frosthaven-manager |
value
value
value
value
value
value
value
Serializable.
value
value
value
value
value
value
value
value
value
value
Serializable.
value
value
value
value
value
value
value
value
value
value
value
value
value
value
Serializable.
procedure
c : condition?
procedure
(selector:condition i) → condition?
i : integer?
procedure
(initiative? v) → boolean?
v : any/c
value
value
expirable-conditions : (setof conditions?)
procedure
(conditions->string cs) → string?
cs : (listof condition?)
value
value
value
procedure
(shuffle-modifier-deck? deck) → boolean?
deck : (listof monster-modifier?)
procedure
(better-modifier a b) → monster-modifier?
a : monster-modifier? b : monster-modifier?
procedure
(worse-modifier a b) → monster-modifier?
a : monster-modifier? b : monster-modifier?
procedure
(absent-from-modifier-deck cards) → (listof monster-modifier?)
cards : (listof monster-modifier?)
7.7 elements
(require frosthaven-manager/elements) | |
package: frosthaven-manager |
value
struct
(struct element-pics (name infused waning unfused consume) #:transparent) name : string? infused : pict? waning : pict? unfused : pict? consume : pict?
procedure
(elements) → (listof element-pics?)
7.8 enum-helpers
(require frosthaven-manager/enum-helpers) | |
package: frosthaven-manager |
syntax
(define-serializable-enum-type id (constant-id ...) enum-option ...)
enum-option = #:omit-root-binding | #:descriptor-name descriptor-id | #:predicate-name predicate-id | #:discriminator-name discriminator-id | #:selector-name selector-id | #:property-maker prop-maker-expr | #:inspector inspector-expr
prop-maker-expr :
(-> uninitialized-enum-descriptor? (listof (cons/c struct-type-property? any/c)))
inspector-expr : inspector?
7.9 icons
(require frosthaven-manager/icons) | |
package: frosthaven-manager |
This module provides various icons that are spliced into ability card texts. All replacements are case insensitive; any numbers or other accompanying text are preserved. The signifier N denotes where a number is expected.

Target N
Target all
+N target(s)

Range N

Push N

Pull N

Move +N
Move -N
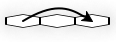
Jump

Teleport
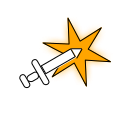
Attack +N
Attack -N
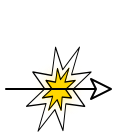
Pierce N
7.10 manager
(require frosthaven-manager/manager) | |
package: frosthaven-manager |
This module reprovides all the bindings from frosthaven-manager/manager/state, frosthaven-manager/manager/ability-decks, frosthaven-manager/manager/modifier-decks, frosthaven-manager/manager/db, frosthaven-manager/manager/elements, frosthaven-manager/manager/loot, frosthaven-manager/manager/round-prompts, frosthaven-manager/manager/transition, and frosthaven-manager/manager/save.
7.10.1 manager/state
(require frosthaven-manager/manager/state) | |
package: frosthaven-manager |
This module provides facilities for manipulating manager-level state.
A creature is identified by its unique creature-id.
Serializable.
struct
(struct monster-group* (active mg) #:transparent) active : (or/c #f monster-number/c) mg : monster-group?
Serializable.
procedure
(creature-is-mg*? c) → any/c
c : creature?
struct
(struct state ( @level @num-players @creatures @type->number-of-cards @loot-deck @num-loot-cards @elements @in-draw? @round @monster-modifier-deck @monster-discard @player-blesses @curses @blesses @modifier @monster-prev-discard @bestiary-path @ability-decks @prompts @type->deck @error-logs)) @level : (obs/c level/c) @num-players : (obs/c num-players/c) @creatures : (obs/c (listof creature?)) @type->number-of-cards : (obs/c (hash/c loot-type/c natural-number/c)) @loot-deck : (obs/c (listof loot-card?)) @num-loot-cards : (obs/c natural-number/c) @elements : (listof (obs/c element-state/c)) @in-draw? : (obs/c boolean?) @round : (obs/c natural-number/c) @monster-modifier-deck : (obs/c (listof monster-modifier?)) @monster-discard : (obs/c (listof monster-modifier?)) @player-blesses : (obs/c (listof monster-modifier?)) @curses : (obs/c (listof monster-modifier?)) @blesses : (obs/c (listof monster-modifier?)) @modifier : (obs/c (or/c #f monster-modifier?)) @monster-prev-discard : (obs/c (or/c #f monster-modifier?)) @bestiary-path : (obs/c (or/c #f path-string?)) @ability-decks : (obs/c (hash/c string? ability-decks?)) @prompts : (obs/c (listof prompt/c)) @type->deck : (maybe-obs/c (hash/c loot-type/c (listof loot-card?))) @error-logs : (obs/c (or/c #f path?))
procedure
(make-state [ @level @num-players @creatures @type->number-of-cards @loot-deck @num-loot-cards @elements @in-draw? @round @monster-modifier-deck @monster-discard @player-blesses @curses @blesses @modifier @monster-prev-discard @bestiary-path @ability-decks @prompts @type->deck] @error-logs) → state? @level : (maybe-obs/c level/c) = (@ 0) @num-players : (maybe-obs/c num-players/c) = (@ 2) @creatures : (maybe-obs/c (listof creature?)) = (@ empty)
@type->number-of-cards : (maybe-obs/c (hash/c loot-type/c natural-number/c)) = (@ (hash)) @loot-deck : (maybe-obs/c (listof loot-card?)) = (@ empty) @num-loot-cards : (maybe-obs/c natural-number/c) = (@ 0)
@elements : (listof (maybe-obs/c element-state/c)) = (make-states '(fire ice air earth light dark)) @in-draw? : (maybe-obs/c boolean?) = (@ #f) @round : (maybe-obs/c natural-number/c) = (@ 1)
@monster-modifier-deck : (maybe-obs/c (listof monster-modifier?)) = (@ (shuffle monster-modifier-deck))
@monster-discard : (maybe-obs/c (listof monster-modifier?)) = (@ empty)
@player-blesses : (maybe-obs/c (listof monster-modifier?)) = (@ empty)
@curses : (maybe-obs/c (listof monster-modifier?)) = (@ monster-curse-deck)
@blesses : (maybe-obs/c (listof monster-modifier?)) = (@ bless-deck) @modifier : (maybe-obs/c (or/c #f monster-modifier?)) = (@ #f)
@monster-prev-discard : (maybe-obs/c (or/c #f monster-modifier?)) = (@ #f) @bestiary-path : (maybe-obs/c (or/c #f path-string?)) = (@ #f)
@ability-decks : (maybe-obs/c (hash/c string? ability-decks?)) = (@ (hash)) @prompts : (maybe-obs/c (listof prompt/c)) = (@ empty)
@type->deck : (maybe-obs/c (hash/c loot-type/c (listof loot-card?))) = (@ standard-loot-deck) @error-logs : (maybe-obs/c (or/c #f path?))
procedure
(state-@env s) → (obs/c env/c)
s : state?
procedure
(state-@info-db s) → (obs/c info-db/c)
s : state?
procedure
(state-@ability-db s) → (obs/c ability-db/c)
s : state?
procedure
(serialize-state s out) → any
s : state? out : output-port?
procedure
(deserialize-state in) → state?
in : input-port?
procedure
(copy-state from to) → any
from : state? to : state?
Warning: sometimes multiple undos are necessary to be coherent. Not all state changes are recorded.
procedure
i : any/c
procedure
(setup-players s) → any
s : state?
procedure
(update-players creatures k f) → (listof creature?)
creatures : (listof creature?) k : any/c f : (-> player? player?)
procedure
(update-monster-groups creatures k f [fn]) → (listof creature?)
creatures : (listof creature?) k : any/c f : (-> monster-group? monster-group?)
fn : (-> (or/c #f monster-number/c) monster-group? (or/c #f monster-number/c)) = (flow 1>)
procedure
(kill-monster s monster-group-id monster-number) → any s : state? monster-group-id : any/c monster-number : monster-number/c
procedure
(update-all-players creatures f) → (listof creature?)
creatures : (listof creature?) f : (-> player? player?)
procedure
(update-all-monster-groups creatures f) → (listof creature?)
creatures : (listof creature?) f : (-> monster-group? monster-group?)
procedure
((update-player-name s) k name) → any
s : state? k : any/c name : string?
procedure
((update-player-max-hp s) k f) → any
s : state? k : any/c f : (-> natural-number/c natural-number/c)
procedure
(creature-initiative ads)
→ (-> creature? (or/c +inf.0 initiative?)) ads : (hash/c string? ability-decks?)
value
=
(or/c (list/c 'set 'from string? 'to string?) (list/c 'monster 'from monster-info? 'to monster-info?) (list/c 'include? monster-number/c 'to boolean?) (list/c 'elite? monster-number/c 'to boolean?) (list/c 'level level/c))
value
add-monster-event/c : contract? = (list/c 'add monster-group?)
value
= (list/c 'remove monster-group?)
procedure
→
(-> (or/c add-monster-event/c remove-monster-event/c) any) s : state?
procedure
(draw-new-card-mid-round-if-needed s set) → any
s : state? set : string?
It is the caller’s responsibility to verify that a monster has been added and needs to potentially trigger a new card.
procedure
(initiative-public? in-draw?) → boolean?
in-draw? : boolean?
procedure
(add-prompt s) → (-> prompt/c any)
s : state?
procedure
((remove-prompt s) i p) → any
s : state? i : natural-number/c p : prompt/c
7.10.2 manager/ability-decks
(require frosthaven-manager/manager/ability-decks) | |
package: frosthaven-manager |
struct
(struct ability-decks (current draw discard) #:transparent) current : (or/c #f monster-ability?) draw : (listof monster-ability?) discard : (listof monster-ability?)
Serializable.
procedure
ad : ability-decks?
procedure
(ability-decks-discard-and-maybe-shuffle ad) → ability-decks?
ad : ability-decks?
procedure
→ (-> (hash/c string? ability-decks?) (hash/c string? ability-decks?)) f : (-> string? ability-decks? ability-decks?)
procedure
ad : ability-decks?
7.10.3 manager/modifier-decks
(require frosthaven-manager/manager/modifier-decks) | |
package: frosthaven-manager |
This module provides facilities for manipulating the modifier deck.
procedure
(reshuffle-modifier-deck s) → any
s : state?
procedure
s : state? card : monster-modifier?
procedure
(draw-modifier s) → (-> any)
s : state?
procedure
(draw-modifier* s keep) → (-> any)
s : state? keep : (-> monster-modifier? monster-modifier? monster-modifier?)
procedure
(do-curse-monster s) → (-> any)
s : state?
procedure
(do-bless-monster s) → (-> any)
s : state?
procedure
(do-bless-player s) → (-> any)
s : state?
procedure
(do-unbless-player s) → (-> any)
s : state?
procedure
(add-monster-modifier s) → (-> monster-modifier? any)
s : state?
procedure
→ (-> exact-nonnegative-integer? any) s : state?
7.10.4 manager/db
(require frosthaven-manager/manager/db) | |
package: frosthaven-manager |
This module provides facilities for manipulating the active monster databases.
procedure
db : path-string? s : state?
procedure
(init-dbs-and-foes db s) → any
db : path-string? s : state?
7.10.5 manager/elements
(require frosthaven-manager/manager/elements) | |
package: frosthaven-manager |
value
element-state/c : contract? = (or/c 'unfused 'infused 'waning)
procedure
(make-states es) → (listof (obs/c element-state/c))
es : (listof any/c)
procedure
(infuse-all es) → any
es : (listof (obs/c element-state/c))
procedure
(consume-all es) → any
es : (listof (obs/c element-state/c))
procedure
(wane-element state) → element-state/c
state : element-state/c
procedure
(transition-element-state state) → element-state/c
state : element-state/c
7.10.6 manager/loot
(require frosthaven-manager/manager/loot) | |
package: frosthaven-manager |
This module provides facilities for manipulating the loot deck.
procedure
(build-loot-deck type->number-of-cards type->deck) → (listof loot-card?) type->number-of-cards : (hash/c loot-type/c natural-number/c) type->deck : (hash/c loot-type/c (listof loot-card?))
The mapping type->number-of-cards maps a loot card type to a number of loot cards of that type. The mapping type->deck specifies which deck cards of that type should be drawn from.
This function assumes, but does not check, that for all types t the number of cards for that type (hash-ref type->number-of-cards t) is less than or equal to the number of loot cards in that type’s deck (length (hash-ref type->deck t)).
procedure
(build-loot-deck! s) → any
s : state?
procedure
((give-player-loot s) k) → any
s : state? k : any/c
procedure
(place-loot-on-bottom s) → any
s : state?
procedure
(player->rewards p num-players level) → (listof string?)
p : player? num-players : num-players/c level : level/c
7.10.7 manager/round-prompts
(require frosthaven-manager/manager/round-prompts) | |
package: frosthaven-manager |
This module provides facilities for manipulating round prompt values.
syntax
(prompt [time rule] ...)
rule = m-expr | even | odd | every n-expr starting-at start-expr
time : time/c
m-expr : natural-number/c
n-expr : natural-number/c
start-expr : natural-number/c
A rule consisting of m-expr means to prompt at round m-expr.
A rule consisting of even means to prompt at every even round.
A rule consisting of odd means to prompt at every odd round.
A rule consisting of every n-expr starting-at start-expr means to prompt at every n-exprth round, starting at the start-exprth round.
procedure
(should-do-prompt? t current-round prompts) → any/c
t : time/c current-round : natural-number/c prompts : (listof prompt/c)
value
value
value
value
procedure
(prompt->string p) → string?
p : prompt/c
7.10.8 manager/transition
(require frosthaven-manager/manager/transition) | |
package: frosthaven-manager |
value
value
7.10.9 manager/save
(require frosthaven-manager/manager/save) | |
package: frosthaven-manager |
procedure
(do-save-game s) → any
s : state?
procedure
(do-load-game s) → any
s : state?
procedure
s : state? p : path-string?
7.11 gui
This collection provides modules for operating GUIs.
7.11.1 gui/common-menu
(require frosthaven-manager/gui/common-menu) | |
package: frosthaven-manager |
value
about-menu-item : (-> (is-a?/c view<%>))
value
issue-menu-item : (-> (is-a?/c view<%>))
value
feature-menu-item : (-> (is-a?/c view<%>))
value
contribute-menu-item : (-> (is-a?/c view<%>))
value
value
value
value
gc-menu-item : (-> (is-a?/c view<%>))
7.11.2 gui/counter
(require frosthaven-manager/gui/counter) | |
package: frosthaven-manager |
7.11.3 gui/elements
(require frosthaven-manager/gui/elements) | |
package: frosthaven-manager |
procedure
@states : (listof (obs/c element-state/c)) es : (listof element-pics?) panel : (unconstrained-domain-> (is-a?/c view<%>)) = hpanel
7.11.4 gui/font
(require frosthaven-manager/gui/font) | |
package: frosthaven-manager |
This module provides helpers for manipulating font objects, as in font%.
procedure
→ (is-a?/c font%) f : (is-a?/c font%)
size : (real-in 0.0 1024.0) = (send f get-size size-in-pixels?) face : (or/c string? #f) = (send f get-face)
family : (or/c 'default 'decorative 'roman 'script 'swiss 'modern 'symbol 'system) = (send f get-family) style : (or/c 'normal 'italic 'slant) = (send f get-style) weight : font-weight/c = (send f get-weight) underlined? : any/c = (send f get-underlined)
smoothing : (or/c 'default 'partly-smoothed 'smoothed 'unsmoothed) = (send f get-smoothing) size-in-pixels? : any/c = (send f get-size-in-pixels) hinting : (or/c 'aligned 'unaligned) = (send f get-hinting)
feature-settings : font-feature-settings/c = (send f get-feature-settings)
font-list : (or/c (is-a?/c font-list%) #f) = (current-font-list)
7.11.5 gui/formula-editor
(require frosthaven-manager/gui/formula-editor) | |
package: frosthaven-manager |
This module provides GUI objects for interactive formula editing.
7.11.6 gui/helpers
(require frosthaven-manager/gui/helpers) | |
package: frosthaven-manager |
procedure
→
position-integer? position-integer? this : (is-a?/c area<%>) top : (is-a?/c area<%>) x : position-integer? y : position-integer?
7.11.7 gui/level-info
(require frosthaven-manager/gui/level-info) | |
package: frosthaven-manager |
procedure
@level : (obs/c level/c) @num-players : (obs/c num-players/c)
procedure
@num-players : (obs/c num-players/c)
7.11.8 gui/level-picker
(require frosthaven-manager/gui/level-picker) | |
package: frosthaven-manager |
procedure
(level-picker #:choose on-choose #:selection selection [ #:label label]) → (is-a?/c view<%>) on-choose : (-> level/c any) selection : (maybe-obs/c level/c) label : (maybe-obs/c maybe-label/c) = #f
7.11.9 gui/loot
(require frosthaven-manager/gui/loot) | |
package: frosthaven-manager |
procedure
(loot-picker @type->cards @type->deck [ #:on-card on-card #:on-deck on-deck]) → (is-a?/c view<%>) @type->cards : (obs/c (hash/c loot-type/c natural-number/c)) @type->deck : (obs/c (hash/c loot-type/c (listof loot-card?)))
on-card : (-> (list/c (or/c 'add 'remove) loot-type/c) any) = void
on-deck : (-> (hash/c loot-type/c (listof loot-card?)) any) = void
This picker allows loading new decks of loot cards and stickering cards on the fly with + 1 stickers. It does not allow removing such stickers; reset the deck to start over.
procedure
(loot-button @loot-deck @num-loot-cards @num-players @players [ #:on-player on-player #:on-top on-top #:on-bottom on-bottom]) → (is-a?/c view<%>) @loot-deck : (obs/c (listof loot-card?)) @num-loot-cards : (obs/c natural-number/c) @num-players : (obs/c num-players/c) @players : (obs/c (listof (cons/c player? any/c))) on-player : (-> any/c any) = void on-top : (-> any) = void on-bottom : (-> any) = void
Additionally, buttons for the top and bottom of the deck trigger the on-top and on-bottom callbacks, which then also close the view.
See Scenario information and loot for how loot-button functions in Frosthaven Manager.
procedure
(loot-preview @loot-deck @num-players) → (is-a?/c view<%>)
@loot-deck : (obs/c (listof loot-card?)) @num-players : (obs/c num-players/c)
7.11.10 gui/manager
(require frosthaven-manager/gui/manager) | |
package: frosthaven-manager |
This module’s main function is to run the Frosthaven Manager. It provides only a single binding:
procedure
(manager s) → (is-a?/c window-view<%>)
s : state?
7.11.11 gui/markdown
(require frosthaven-manager/gui/markdown) | |
package: frosthaven-manager |
procedure
(markdown-text @content [ #:min-size min-size #:stretch stretch #:margin margin #:inset inset #:style style]) → (is-a?/c view<%>) @content : (maybe-obs/c (or/c string? path?)) min-size : (maybe-obs/c size/c) = '(#f #f) stretch : (maybe-obs/c stretch/c) = '(#t #t) margin : (maybe-obs/c margin/c) = '(0 0) inset : (maybe-obs/c margin/c) = '(5 5)
style :
(listof (one-of/c 'no-border 'control-border 'combo 'no-hscroll 'no-vscroll 'hide-hscroll 'hide-vscroll 'auto-vscroll 'auto-hscroll 'resize-corner 'deleted 'transparent)) = '(no-hscroll)
Paragraphs;
HTML comments;
Hyperlinks;
Blockquotes;
Unordered and ordered lists;
Horizontal rules;
Bold, italic, and code styles;
and six levels of headings.
Any string
Any expression tagged !HTML-COMMENT, the tag for HTML comments
Any expression tagged a
Any expression tagged blockquote
Any expression tagged ul
Any expression tagged ol
Any expression tagged li
Any expression tagged hr
Any expression tagged p
Any expression tagged strong
Any expression tagged em
Any expression tagged code
Any expression tagged h1
Any expression tagged h2
Any expression tagged h3
Any expression tagged h4
Any expression tagged h5
Any expression tagged h6
Note that Markdown technically requires 4 spaces or a single tab as leading indent for nesting lists and other blocks; while many Markdown implementations (such as those used on GitHub) are more lenient, the implementation backing markdown-text is stricter on this point.
7.11.12 gui/mixins
(require frosthaven-manager/gui/mixins) | |
package: frosthaven-manager |
procedure
→ (make-mixin-contract top-level-window<%>) out : (-> (-> any) any)
procedure
→ (make-mixin-contract top-level-window<%>) proc : (-> any)
7.11.13 gui/monster-modifier
(require frosthaven-manager/gui/monster-modifier) | |
package: frosthaven-manager |
procedure
(modify-monster-deck-menu-item @cards [ #:on-add on-add #:on-remove on-remove] #:on-shuffle on-shuffle) → (is-a?/c view<%>) @cards : (obs/c (listof monster-modifier?)) on-add : (-> monster-modifier? any) = void on-remove : (-> exact-nonnegative-integer? any) = void on-shuffle : (-> any)
procedure
(favors-dialog @cards [ #:on-add on-add #:on-remove on-remove] #:on-shuffle on-shuffle) → (is-a?/c window-view<%>) @cards : (obs/c (listof monster-modifier?)) on-add : (-> monster-modifier? any) = void on-remove : (-> exact-nonnegative-integer? any) = void on-shuffle : (-> any)
procedure
(card-swapper @cards [ #:on-add on-add #:on-remove on-remove]) → (is-a?/c view<%>) @cards : (obs/c (listof monster-modifier?)) on-add : (-> monster-modifier? any) = void on-remove : (-> exact-nonnegative-integer? any) = void
When a card is added via the <= arrow, calls on-add with the card. When a card is removed via the => arrow, calls on-remove with the cards index.
7.11.14 gui/monsters
(require frosthaven-manager/gui/monsters) | |
package: frosthaven-manager |
procedure
(single-monster-picker info-db @initial-level [ #:on-change on-change #:unavailable unavailable]) → (is-a?/c view<%>) info-db : info-db/c @initial-level : (obs/c level/c) on-change : (-> single-monster-event/c any) = void unavailable : (set/c string?) = empty
procedure
(simple-monster-group-view @mg) → (is-a?/c view<%>)
@mg : (obs/c monster-group?)
procedure
(monster-group-view @mg @ability-deck @monster-num @env [ #:on-select on-select #:on-condition on-condition #:on-hp on-hp #:on-kill on-kill #:on-new on-new #:on-swap on-swap #:on-move-ability-card on-move-ability-card #:on-max-hp on-max-hp] #:on-change-level on-change-level #:on-update arbitrary-update) → (is-a?/c view<%>) @mg : (obs/c monster-group?) @ability-deck : (obs/c ability-decks?) @monster-num : (obs/c (or/c #f monster-number/c)) @env : (obs/c env/c) on-select : (-> (or/c #f monster-number/c) any) = void
on-condition : (-> monster-number/c condition? boolean? any) = void on-hp : (-> monster-number/c (-> number? number?) any) = void on-kill : (-> monster-number/c any) = void on-new : (-> monster-number/c boolean? any) = void on-swap : (-> (or/c 'all monster-number/c) any) = void on-move-ability-card : (-> any) = void
on-max-hp : (-> (-> (or/c 'normal 'elite) natural-number/c number?) any) = void on-change-level : (-> level/c any) arbitrary-update : (-> (-> monster-group? monster-group?) any)
on-select is given a new monster number when one is selected in the detailed view, or #false if there are none.
on-condition is given a monster number, condition, and either #true or #false to indiciate whether the condition should be applied or removed.
on-hp is given a monster number and a procedure to update the monsters monster-current-hp.
on-kill is invoked with a monster number when that monster is killed.
on-new is invoked with a monster number and #true if the monster is elite or #false otherwise for a newly added monster.
on-swap is invoked with 'all if all monsters should be swapped by swap-monster-group-elites, or with a monster number if only that monster should be swapped by swap-monster-elite.
on-move-ability-card is invoked when the top of the ability draw pile should be moved to the bottom using the ability deck previewer.
on-max-hp is invoked when adjusting all maximum HP of the group. The given procedure computes new maximum HP values as for monster-group-change-max-HP.
on-change-level is invoked when adjusting the group’s level with the new level.
The arbitrary-update callback is invoked with a function that computes a new monster-group?; it is intended to update @mg for more complicated events that are logically a single step.
procedure
(db-view @info-db @ability-db @monster-groups) → (is-a?/c view<%>) @info-db : (obs/c info-db/c) @ability-db : (obs/c ability-db/c) @monster-groups : (obs/c (listof monster-group?))
Any pre-set monster groups will also be shown.
procedure
(add-monster-group @info-db @initial-level @monster-names @env [ #:on-group on-group]) → any @info-db : (obs/c info-db/c) @initial-level : (obs/c level/c) @monster-names : (obs/c (set/c string? #:cmp 'dont-care #:kind 'dont-care)) @env : (obs/c env/c) on-group : (-> monster-group? any) = void
7.11.15 gui/number-players
(require frosthaven-manager/gui/number-players) | |
package: frosthaven-manager |
procedure
(number-players-picker #:choose on-choose #:selection selection [ #:label label]) → (is-a?/c view<%>) on-choose : (-> level/c any) selection : (maybe-obs/c level/c) label : (maybe-obs/c maybe-label/c) = "Number of Players"
7.11.16 gui/player-info
(require frosthaven-manager/gui/player-info) | |
package: frosthaven-manager |
procedure
→ (is-a?/c view<%>) @player : (obs/c player?) on-condition : (-> (list/c condition? boolean?) any) = void on-hp : (-> (-> number? number?) any) = void on-xp : (-> (-> number? number?) any) = void on-initiative : (-> number? any) = void arbitrary-update : (-> (-> player? player?) any) add-summon : (-> string? positive-integer? any) update-summon-hp : (-> natural-number/c (-> number? number?) any) update-summon-condition : (-> natural-number/c (list/c condition? boolean?) any) kill-summon : (-> natural-number/c any)
The summon callbacks are given the summon number, a list index, to indicate which summon to update. Adding a summon is done by name and max HP.
The arbitrary-update callback is invoked with a function that computes a new player?; it is intended to update @player for more complicated events that are logically a single step.
7.11.17 gui/render
(require frosthaven-manager/gui/render) | |
package: frosthaven-manager |
parameter
(current-renderer) → (or/c #f renderer?)
(current-renderer r) → void? r : (or/c #f renderer?)
= #f
(define root (render ...)) (current-renderer root)
This will not affect multiple applications built and run separately that use this library, since they’re in separate processes completely.
procedure
(render/eventspace tree [ #:parent parent #:eventspace es]) → renderer? tree : (is-a?/c view<%>) parent : (or/c #f renderer?) = #f es : eventspace? = (current-eventspace)
Pass a new eventspace created with make-eventspace to separate the rendered tree and corresponding current-renderer from other applications.
This can be used to group windows in an application together, but note that subsequent calls with the same es will override that eventspace’s handler thread’s current-renderer.
For a short-lived window that should tear down the eventspace on closure, combine with with-closing-custodian/eventspace.
syntax
(with-closing-custodian/eventspace e ...+)
syntax
syntax
syntax
closing-custodian is a new custodian that manages closing-eventspace.
closing-eventspace is a new eventspace managed by closing-custodian.
close-custodian-mixin is a new mixin for top-level-window<%>s that causes closing-custodian to shutdown after the corresponding window is closed.
(require racket/gui/easy) (define main-es (make-eventspace)) (render/eventspace #:eventspace main-es (window #:title "A" (text "A"))) (define aux-es (with-closing-custodian/eventspace (render/eventspace #:eventspace closing-eventspace (window #:mixin close-custodian-mixin #:title "B" (text "B"))) closing-eventspace)) (sync main-es) ;; wait until window A is closed (eventspace-shutdown? aux-es) ;; true if window B was closed first
7.11.18 gui/rewards
(require frosthaven-manager/gui/rewards) | |
package: frosthaven-manager |
This module contains views for end-of-scenario rewards.
procedure
(player-rewards-view @num-players @level @players [ #:mixin mix]) → (is-a?/c view<%>) @num-players : (obs/c num-players/c) @level : (obs/c level/c) @players : (obs/c (listof player?)) mix : (make-mixin-contract top-level-window<%>) = values
7.11.19 gui/rich-text-display
(require frosthaven-manager/gui/rich-text-display) | |
package: frosthaven-manager |
This module provides a view-based rich text display, suitable for certain replacements of text.
procedure
(rich-text-display @content [ #:font @font #:min-size @min-size #:stretch @stretch #:margin @margin #:inset @inset #:style style]) → (is-a?/c view<%>)
@content :
(maybe-obs/c (listof (or/c string? pict? pict/alt-text? newline?))) @font : (maybe-obs/c (is-a?/c font%)) = normal-control-font @min-size : (maybe-obs/c size/c) = '(#f #f) @stretch : (maybe-obs/c stretch/c) = '(#t #t) @margin : (maybe-obs/c margin/c) = '(0 0) @inset : (maybe-obs/c margin/c) = '(5 5)
style :
(listof (one-of/c 'no-border 'control-border 'combo 'no-hscroll 'no-vscroll 'hide-hscroll 'hide-vscroll 'auto-vscroll 'auto-hscroll 'resize-corner 'deleted 'transparent)) = '(no-hscroll)
Contents are selectable and copyable with the usual keyboard shortcuts, and can also be selected with mouse. Contents are automatically reflowed.
7.11.19.1 Rich Text Model
(require (submod frosthaven-manager/gui/rich-text-display model)) |
struct
(struct pict/alt-text (p alt-text))
p : pict? alt-text : string?
procedure
(scale-icon p) → pict?
p : pict?
7.11.20 gui/round-number
(require frosthaven-manager/gui/round-number) | |
package: frosthaven-manager |
This module contains GUI components for interacting with the round number.
procedure
(round-number-modifier @round [ #:new-round-number new-round-number]) → (is-a?/c window-view<%>) @round : (obs/c natural-number/c)
new-round-number : (-> (-> natural-number/c natural-number/c) any) = void
7.11.21 gui/round-prompts
(require frosthaven-manager/gui/round-prompts) | |
package: frosthaven-manager |
This module contains GUI utilities for frosthaven-manager/manager/round-prompts.
procedure
(prompts-input-view @prompts [ #:on-add on-add #:on-remove on-remove]) → (is-a?/c view<%>) @prompts : (obs/c (listof prompt/c)) on-add : (-> prompt/c any) = void on-remove : (-> natural-number/c prompt/c any) = void
procedure
(manage-prompt-menu-item @prompts [ #:on-add on-add #:on-remove on-remove]) → (is-a?/c view<%>) @prompts : (obs/c (listof prompt/c)) on-add : (-> prompt/c any) = void on-remove : (-> natural-number/c prompt/c any) = void
procedure
(do-round-prompt t round) → any
t : time/c round : natural-number/c
7.11.22 gui/server
(require frosthaven-manager/gui/server) | |
package: frosthaven-manager |
7.11.23 gui/stacked-tables
(require frosthaven-manager/gui/stacked-tables) | |
package: frosthaven-manager |
procedure
(stacked-tables [ #:topleft? topleft? #:panel panel] @data final-view column1 column-spec ...) → (is-a?/c view<%>) topleft? : boolean? = #t panel : (-> (is-a?/c view<%>) ... (is-a?/c view<%>)) = hpanel @data : (obs/c (vectorof any/c)) final-view : (-> (obs/c (or/c #f any/c)) (is-a?/c view<%>)) column1 : column? column-spec : column?
The stack of tables is determined by column1 and each column-spec. The first is always column1.
Starting with @data and column1, a table is added to the stack. The table’s title is given by column-title. The labels for the items in the table come from applying column-entry->label to the values in the data. When a value is selected, the data for the next table and column-spec is produced by column-entry->next on the selection. This value is automatically wrapped in vector as needed.
This process continues, adding tables to the stack whose data depends on previous data and selections, until the final table and column-spec are added. The final selection, which is not automatically vectorized, is given to final-view. The resulting view is also added to the stack.
struct
title : string? entry->label : (-> any/c string?) entry->next : (-> any/c (or/c any/c (vectorof any/c)))
A note about column-entry->next: you almost certainly want to return a vector for all but (possibly) the last column. Intermediate columns likely have multiple choices. As a convenience, when there is only one, you may omit the vector. For the final column, you likely want to omit the vector unless the selected data is one: the data here is the final selection, of which there should probably be one.
7.11.24 gui/static-table
(require frosthaven-manager/gui/static-table) | |
package: frosthaven-manager |
procedure
(static-table columns num-rows entry->columns [ #:index->entry index->entry #:entry->value entry->value #:selection @selection #:widths widths]) → (is-a?/c view<%>) columns : (listof label-string?) num-rows : natural-number/c entry->columns : (listof (-> any/c any/c)) index->entry : (-> natural-number/c natural-number/c) = values entry->value : (-> natural-number/c any/c) = values
@selection :
(maybe-obs/c (or/c #f exact-nonnegative-integer? (listof exact-nonnegative-integer?))) = #f
Summarizing: each row is indexed by a natural number in the range [0,num-rows). An entry is computed by index->entry. A value is computed from the entry by entry->value. From this value, functions in entry->columns compute the elements of the row.
The selection is determined by @selection as with table.
The column widths are calculated automatically based on columns, or are provided as widths.
7.11.25 gui/table
(require frosthaven-manager/gui/table) | |
package: frosthaven-manager |
procedure
(make-preview-rows xs n #:reveal reveal #:hide hide) → (vectorof (vectorof string?)) xs : list? n : (or/c 'all natural-number/c) reveal : (-> any/c (vectorof string?)) hide : (-> any/c (vectorof string?))
If n is 'all or greater than the length of xs, all elements are considered revealed.
Rows correspond to the notion of rows or entries in table.
7.12 files
(require frosthaven-manager/files) | |
package: frosthaven-manager |
procedure
(get-file/filter message filter) → (or/c path? #f)
message : label-string? filter : (list/c string? string?)
procedure
(put-file/filter message filter [ directory file]) → (or/c path? #f) message : label-string? filter : (list/c string? string?) directory : path-string? = #f file : path-string? = #f
7.13 bestiary
This module implements the bestiary language. See Programming a Scenario and frosthaven-manager/bestiary for more information.
7.14 monster-db
(require frosthaven-manager/monster-db) | |
package: frosthaven-manager |
See Programming a Scenario for more information on custom monster databases.
value
value
procedure
(datums->dbs xs) →
info-db/c ability-db/c xs : (listof any/c)
procedure
(get-dbs db-file) →
info-db/c ability-db/c db-file : path-string?
value
7.15 parsers
This collection contains parsers that support various #langs.
7.15.1 parsers/foes
(require frosthaven-manager/parsers/foes) | |
package: frosthaven-manager |
This module contains parsers for #lang frosthaven-manager/foes. See Programming a Scenario for more details.
procedure
(parse-foes src in #:syntax? syn?) → (or/c syntax? foes/pc)
src : any/c in : input-port? syn? : any/c
value
= (list/c (cons/c 'import (listof string?)) (cons/c 'info (listof monster-info?)) (cons/c 'ability (listof monster-ability?)) (cons/c 'foe (listof foe/pc)))
value
= (list/c string? string? numbering/pc (listof spec/pc))
value
= (hash/c num-players/c monster-type/pc #:immutable #t)
value
numbering/pc : flat-contract? = (or/c "ordered" "random" #f)
value
= (or/c "absent" "normal" "elite")
7.15.2 parsers/formula
(require frosthaven-manager/parsers/formula) | |
package: frosthaven-manager |
This module contains parsers for arithmetic formulas over addition, subtraction, multiplication, division, rounding, and a limited set of variables. The parse result is a function from an environment of variables to a number.
procedure
(parse-expr in) → expr/pc
in : string?
7.15.3 parsers/monster
(require frosthaven-manager/parsers/monster) | |
package: frosthaven-manager |
This module contains parsers for #lang frosthaven-manager/bestiary. See Programming a Scenario for more details.
procedure
(parse-bestiary src in #:syntax? syn?)
→ (or/c syntax? bestiary/c) src : any/c in : input-port? syn? : any/c
value
=
(list/c (cons/c 'import (listof string?)) (cons/c 'info (listof monster-info?)) (cons/c 'ability (listof monster-ability?)))
value
value
value
import-monsters/p : (parser/c char? (list/c 'import string?))
value
procedure
(bestiary-dupes xs) →
(or/c #f (listof string?)) (or/c #f (listof string?)) xs : (listof any/c)
7.16 observable-operator
(require frosthaven-manager/observable-operator) | |
package: frosthaven-manager |
In addition to the shorthands below, this module exports define/obs, @, :=, and λ:= from racket/gui/easy/operator and everything from qi via frosthaven-manager/curlique.
7.17 pp
This collection holds pretty printers based on pretty-expressive.
7.17.1 pp/bestiary
(require frosthaven-manager/pp/bestiary) | |
package: frosthaven-manager |
This module pretty-prints bestiary files. It can be run as a program with
racket -l- frosthaven-manager/pp/bestiary
to format standard in or a provided file to standard out. Use --help for more options.
procedure
(pretty-bestiary bestiary [ #:lang-line? lang-line?]) → pretty:doc? bestiary : bestiary/c lang-line? : any/c = #t
The bestiary must not contain any pict values, so it composes best with parse-bestiary.
7.18 qi/list2hash
(require frosthaven-manager/qi/list2hash) | |
package: frosthaven-manager |
syntax
maybe->key =
| #:->key ->key-flo maybe->value =
| #:->value ->value-flo
procedure
(list->hash xs [ #:->key ->key #:->value ->value]) → hash? xs : list? ->key : (-> any/c any/c) = identity ->value : (-> any/c any/c) = identity
7.19 qi/utils
(require frosthaven-manager/qi/utils) | |
package: frosthaven-manager |
procedure
(list-remove xs i) →
list? any/c xs : list? i : natural-number/c
7.20 rich-text-helpers
(require frosthaven-manager/rich-text-helpers) | |
package: frosthaven-manager |
This module provides helpers for converting values to the rich text model of (submod frosthaven-manager/gui/rich-text-display model). In particular, these helpers work with functions that take single values to lists which are intended to be spliced into the resulting model list.
syntax
(match-loop input-expr [match-pattern body-expr ... result-expr] ...)
result-expr : list?
> (define (f x) (match-loop x [(regexp #rx"^(.*)body(.*)$" (list _ prefix suffix)) (list prefix 42 suffix)])) > (f "begin; body 1; body 2; body 3; end") '("begin; " 42 " 1; " 42 " 2; " 42 " 3; end")
A major difference from something like regexp-replace* or regexp-replaces is that the inputs and replacements can be arbitrary values; this codifies the "replace all" loop. Many uses include the same kind of prefix/suffix matching seen in the example above, but the algorithm is more general and can find newly generated matches in result-expr. Take care to avoid generating an infinite loop by unconditionally placing a new match in the result.
7.21 server
(require frosthaven-manager/server) | |
package: frosthaven-manager |
procedure
(launch-server s send-event) →
string? (-> any) s : state? send-event : procedure?
Returns the server address (on a best-guess basis) and a stop procedure that stops the server when called.
7.22 syntax
The modules in this collection provide helpers for macros, syntax, and languages.
7.22.1 syntax/module-reader
(require frosthaven-manager/syntax/module-reader) | |
package: frosthaven-manager |
This expander language wraps syntax/module-reader by assuming a specific reading protocol.
This module does not have a reader of its own, so should be used with module or #lang s-exp.
syntax
(#%module-begin expander-mod-path [parser-id from parser-mod-path])
#lang s-exp frosthaven-manager/syntax/module-reader frosthaven-manager/foes [parse-foes from frosthaven-manager/parsers/foes]
#lang racket (module reader frosthaven-manager/syntax/module-reader frosthaven-manager/foes [parse-foes from frosthaven-manager/parsers/foes])
The semantics are as follows. The resulting module satisfies the language reader extension protocol from Reading via an Extension via syntax/module-reader with a few specifications. The expander-mod-path is used as in syntax/module-reader to determine the module-path for the initial bindings of modules produced by the reader. The parser-id, which must be provided by parser-mod-path, is assumed to parse the whole body as with the #:whole-body-readers? keyword for syntax/module-reader. In addition, it should support the following protocol: the parser accepts 2 positional arguments. The first is the same name-value as read-syntax; the second is the same input port as for read and read-syntax with line-counting enabled. Then it must accept a keyword option #:syntax?, whose value is a boolean indicating whether or not to produce a syntax object.
Examples of valid parsers include parse-foes and parse-bestiary.
7.22.2 syntax/monsters
(require frosthaven-manager/syntax/monsters) | |
package: frosthaven-manager |
syntax
(make-dbs (provide info-db-id ability-db-id) (import import-mod-path ...) (info monster-info ...) (ability monster-ability ...))
monster-info : monster-info?
monster-ability : monster-ability?
Each import-mod-path is expected to provide the same info-db-id and ability-db-id.
The provide keyword in the provide specification is recognized by binding and must be the same as the one from racket/base. The import, info, and ability keywords are recognized by datum identity.
procedure
(imports->dbs import-paths)
→
(listof info-db/c) (listof ability-db/c) import-paths : (listof string?)
procedure
(check-monsters-have-abilities imported-info-dbs imported-ability-dbs infos actions) → boolean? imported-info-dbs : (listof info-db/c) imported-ability-dbs : (listof ability-db/c) infos : (listof monster-info?) actions : (listof monster-ability?)
procedure
(check-monsters-have-abilities-message imported-info-dbs imported-ability-dbs infos actions) → string? imported-info-dbs : (listof info-db/c) imported-ability-dbs : (listof ability-db/c) infos : (listof monster-info?) actions : (listof monster-ability?)
procedure
(check-foes-have-monsters imported-info-dbs infos foes) → boolean? imported-info-dbs : (listof info-db/c) infos : (listof monster-info?) foes : (listof foe/pc)
procedure
(check-foes-have-monsters-message imported-info-dbs infos foes) → string? imported-info-dbs : (listof info-db/c) infos : (listof monster-info?) foes : (listof foe/pc)